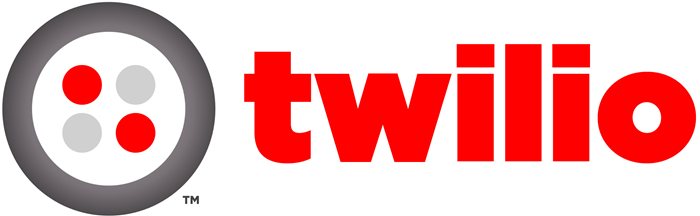
Send a Text Message with Twilio using ASP.NET
Posted on Dec 4, 2013 by
Paul WhiteI recently did a little integration with Twillio for one of my client's
websites. Along the way I had some learning experiences that I thought would be helpful to others. I found Twilio's Sample code to be a little confusing, and the third party libraries and classes did not work for me.
I am the kind of developer that likes to keep things simple. I don't like to reply on classes, or third party libraries that wrap the Twilio code. So after much trial and error I figured out a straight forward way to send a text message using Twilio and
ASP.net using a single function call.
Sending a Text Message using Twilio and ASP.NET
<%@ Import Namespace="System" %>
<%@ Import Namespace="System.IO" %>
<%@ import Namespace="System.Diagnostics" %>
<%@ import Namespace="System.Data" %>
<%@ import Namespace="System.Net" %>
<%@ import Namespace="System.Xml" %>
<%@ import Namespace="System.Data.Odbc" %> <script language="C#" runat="server">
void doit(Object Src, EventArgs E)
sendTwilio("1231231234","This is just a test");
void sendTwilio(string sendto, string message)
string accountSid = "Get this from Twilio";
string authToken = "Get this from Twilio";
sendto="+1"+sendto;
string sendfrom="+1 and your 10 digit Phone number with Twilio";
string url="URL you want status updates to be posted to";
WebRequest request = WebRequest.Create ("https://api.twilio.com/2010-04-01/Accounts/"+accountSid+"/Messages");
request.Method = "POST";
request.Credentials = new NetworkCredential(accountSid,authToken);
string postData = "From="+sendfrom+"&To="+sendto+"&Body="+message+"&StatusCallback="+Server.UrlEncode(url);
byte byteArray = Encoding.UTF8.GetBytes (postData);
request.ContentType = "application/x-www-form-urlencoded";
request.ContentLength = byteArray.Length;
Stream dataStream = request.GetRequestStream ();
dataStream.Write (byteArray, 0, byteArray.Length);
dataStream.Close ();
WebResponse response = request.GetResponse ();
dataStream = response.GetResponseStream ();
StreamReader reader = new StreamReader (dataStream);
string responseFromServer = reader.ReadToEnd ();
// Clean up the streams.
reader.Close ();
dataStream.Close ();
response.Close ();
</script>
<form runat="server">
<asp:Button ID="but1" runat="server" Text="doit" OnClick="doit"/>
</form>
Why Use Twilio vs Email Gateways?
Email Gateways are when you take the 10 digit number and then add the carriers domain ( Example Tmobile is tmomail.net ) at the end to send an email that is converted into a text message. For small lists this works fine, but there are some limitations. Carriers are more likley to block email gateway messages since its free and therefore attracts abuse. The second is Email Gateways don't always get prioritized sending. Twilio messages on the other hand are instant with no delays. Twilio is also relatively cheap. About 1 cent per message. So it will cost you about $10 to send a message to a list of 1000. The other reason Twilio is better is because when people respond to your messages, they come back in a easy to read format. Email Gateways are not always this clean. Often times they use base64 encoding which makes parsing your reply and stop requests very tedious. Twilio can also be setup to send the reply messages to a script for processing. Twilio is a Developer focused system. Once you get an understanding of the APIs and how to work with them, you will start to imagine ways you can streamline communication for your sites and your client's sites.
Twilio Limitations
Before you let you imagination run wild envisioning massive texting systems to spam people's
Cell Phones. Its important you understand the limitations of Twilio. First the sending rate of each Phone number you have with Twilio is limited to 1 Text Message per second. So this means you can send no more than 3600 Text Messages per hour. But each carrier throttles messages differently. The Tech at Twilio said anything more than 250 Messages per day is likely to be blocked by the carriers. So as long as your List of numbers does not have more than 250 Numbers from any one carrier you are fine. The problem is most of the time you don't know what carrier the numbers you have reside at, so its a gamble. To overcome this you can buy multiple numbers from Twilio, then you round robin them as you blast them out.
Discussion
No Comments have been submitted